Essential Software Design Patterns Every Developer Should Know
Kris Nicolaou, March 10, 2023
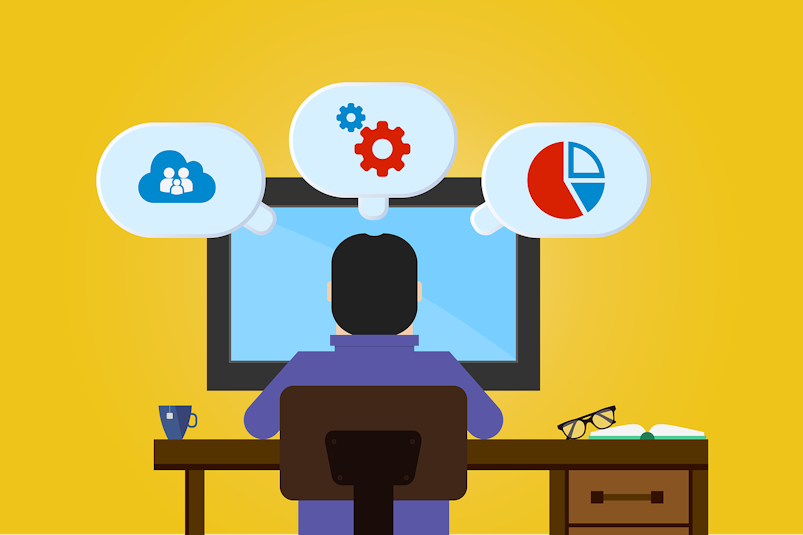
Software development has become more critical than ever in today's digital world. Understanding specific design patterns can make all the difference in creating successful applications. According to a recent report, the software market expects to reach $321.60 billion by 2023, which shows how vital these patterns are for developers today.
The same report also says that enterprise software alone accounts for one-third of the market share. It is estimated to reach $130.10 billion in the same year. This immense potential for software development has seen many developers join the industry, and knowledge about design patterns is essential to success.
This article will discuss four fundamental software design patterns developers should know to create robust and reliable applications. From object-oriented programming strategies to different software architectures, we'll provide an overview of each pattern for you to better understand their importance and application within the software development process.
Key Takeaways
- Facade design patterns hide the complexity of code. Instead, they provide a more intuitive and readable interface for the user. With these patterns, users can enjoy the software more effectively and enjoyably.
- Adapter design patterns let you connect compatible interfaces. This opens up possibilities for far more effective and efficient software.
- Writing clean code is an excellent way to pass on the chain of responsibility to other programmers who wish to continue object-oriented programming projects. Having a clean code can let you fix design problems in the future, even if you hire beginners.
What are software design patterns?
Software design patterns are a set of design principles that guide software development. They help to ensure that code is structured and organized, making it easier to read, maintain and debug. You can also use these patterns to improve the performance and scalability of applications and make them more resilient and secure.
For example, object-oriented programming (OOP) uses design patterns to create reusable solution components and objects, allowing multiple programmers to work on the same software project. Similarly, software architecture includes architectural patterns that you can use to create a robust and efficient application.
These design patterns help programmers create better software faster, allowing them to focus on the core elements of their software development projects rather than worrying about the code. So without further delay, let's look at four essential software design patterns all programmers should know.
Creational Design Patterns
Creational patterns create objects and set up relationships between them. These patterns focus on the instantiation process of objects and can be used to solve the issue of object creation in an efficient and maintainable way. These design patterns are beneficial when dealing with complex object structures, as they can help manage the construction process and reduce coupling between classes.
Here are creational design patterns you can use on your project.
Singleton design patterns
Singleton patterns ensure the creation of only one instance of a particular object. This pattern ensures that all parts of the application use the same data, ensuring changes applied to the data are across all components. It also allows for better control over system resources, as creating multiple copies of the same object is unnecessary.
Furthermore, with the singleton design pattern, developers can gain better control of system resources and allow for more efficient debugging and object creation. It's an effective way to ensure your application remains robust and reliable.
Factory design patterns
Factory patterns provide an interface for creating objects. This design allows for more control and flexibility in the creation process, as it can create objects based on specific criteria or parameters. Factory methods also help to reduce dependencies between objects, making them easier to maintain and modify over time.
Additionally, this pattern is a great way to improve code readability and maintainability as it provides a structure for object creation. It also makes adding new objects or modifying existing ones easier without making code base changes.
Builder design patterns
Constructing objects from multiple parts requires using builder design patterns. This pattern allows for creating complex objects, using steps reusable in multiple ways. Breaking down the construction process into smaller parts helps reduce repetition and simplify code as each section is combined.
Builder design patterns also make it easier to modify existing objects, as each part of the construction is individually adjustable. This design allows for greater flexibility and control over building an object thanks to its elements of reusable object-oriented software architecture. Additionally, it makes it easier to debug code, as errors are less likely to occur when only one part of the object needs to be changed.
Structural Design Patterns
Structural design patterns define the structure and organization of objects and classes. These patterns address relationships between entities in a maintainable, extensible, and reusable way. Structural design patterns help to reduce the complexity of an application's architecture and make it easier for developers to work with.
These design principles focus on the composition of objects and classes to form larger structures and relationships within a software system. These patterns solve problems related to object relationships and interactions and help developers create more flexible and reusable code.
Here are the different structural design patterns you can choose from:
Adapter design patterns
Adapter design patterns can convert the interface of one class into another. This pattern allows previously incompatible objects to work together without significant modifications or changes. This pattern is also helpful in creating a bridge between two existing systems or components, allowing them to interact and efficiently exchange data.
Adapter design patterns can reduce code complexity by providing an abstraction layer between different system parts. This design makes it easier for developers to maintain and debug code, as only one component needs to be changed when something goes wrong.
Additionally, these patterns provide greater flexibility in designing applications, allowing components from disparate sources to work together seamlessly.
Decorator design patterns
Decorator design patterns can extend or modify the functionality of an object without affecting the underlying structure. This pattern allows adding additional features or behaviors without substantially changing the existing code. Using this pattern allows developers to add more functionality to an object without having to rewrite any existing code.
Decorator design patterns are a great way to add functionality to existing objects or classes without making any drastic changes. They allow developers to easily extend existing code's capabilities by attaching additional functionality.
These design patterns are also helpful in creating more complex objects, as you can use them to combine multiple components into one. That makes it easier to maintain code and avoid repetition, as the same components can be used differently without writing multiple versions of the same code. With these patterns, developers can create more feature-rich applications with less effort.
Facade design patterns
Facade design patterns provide a unified interface to a set of individual subsystems. This pattern allows easier access to the system's various components, simplifying the overall structure and eliminating unnecessary complexity. Using this pattern, developers can create more modular code that is easier to maintain and extend over time.
The facade design patterns also provide flexibility, allowing developers to work independently with different system parts. This pattern makes it easier for developers to make changes without modifying other code sections. Additionally, this pattern can reduce dependencies between different subsystems, allowing for more robust and efficient applications.
This design pattern is excellent if you wish to change some parts of the code without worrying so much that the whole system might be brick.
Behavioral Design Patterns
Behavioral design patterns define object interactions, allowing more efficient communication and data exchange. These patterns help minimize dependencies between objects, resulting in easier-to-maintain and extended code.
Additionally, these patterns focus on algorithms and assignments of responsibility, making it possible for teams to manage complex projects better. These patterns also help prevent code repetition, making it easier for developers to reuse existing code. These design patterns provide greater flexibility when designing applications, allowing for more robust and efficient architectures.
Observer design patterns
Observer patterns are types of behavioral patterns used to allow objects to receive notifications when an event occurs within the system. This pattern makes it possible to notify one object when another item changes, allowing for more efficient communication and data exchange.
Additionally, this pattern helps reduce dependencies between different objects, as each object does not need to be aware of the others' inner workings. This design makes it easier for developers to maintain and extend code as they only have to change one component when something goes wrong.
Strategy design patterns
Using strategy design patterns to centralize and simplify algorithms allows them to be more easily changed and maintained. This pattern provides a defined set of steps that you can use to complete a task while also maximizing code reuse and minimizing complexity.
Additionally, these design patterns promote flexibility, as they allow developers to switch between different algorithms depending on the specific needs of an application. That makes it easier for teams to quickly and easily adapt their code according to changes in requirements.
Template method design patterns
Template method design patterns are behavioral patterns that provide an abstract framework for solving a problem while allowing subclasses to define their implementation. This pattern helps reduce code duplication, allowing developers to share standard code between different classes without having to rewrite the entire algorithm.
Additionally, this pattern can reduce application complexity, simplifying the overall structure and minimizing object dependencies. That makes it easier for developers to update and change code as they no longer need to worry about breaking existing functionality.

Architectural Patterns
Architectural patterns define the overall structure of an application and how its components interact with one another. These patterns help developers create more modular code that is easier to maintain, extend, and debug. These design patterns also provide greater scalability, allowing developers to easily add new features and functionality without rewriting existing code.
Here are some architectural patterns you can use for your software development process:
Model-view controller (MVC)
Model-View-Controller (MVC) is an architectural pattern that helps developers separate an application's presentation, data, and business logic. It allows developers to create modular code that is easier to maintain, extend and debug.
MVC promotes better separation of concerns by allowing components to focus on specific tasks, making updating easier for teams. It also helps to create a cleaner overall structure, as each component is separate and independent.
Additionally, it allows code to be reused across different applications, making development more efficient and cost-effective. Finally, MVC makes it easier for developers to test their code since the application's components are isolated. MVC reduces the complexity and time spent on the software development process.
Model-view-view-model (MVVM)
Model-view-view-model (MVVM) is an architectural pattern that builds on the principles of MVC by separating the presentation layer from the business logic. It allows developers to create modular code with well-defined responsibilities, promoting better separation of concerns and making it easier for teams to maintain, extend, and debug code.
VVM enables developers to easily create data bindings between different components, allowing them to quickly and efficiently update the UI when data changes occur. MVVM helps promote better user experiences as users can receive immediate feedback on changes.
Microservices
Microservices are an architectural pattern used to create distributed applications composed of more minor, independent services. This pattern helps to reduce complexity by breaking down large applications into smaller and more manageable sizes that are independently developed and deployed.
Additionally, it enables teams to quickly scale and update components without reconfiguring the entire system. A microservices architecture also helps promote better reusability, as developers can create and reuse services across multiple applications. This architectural pattern makes it easier for teams to adapt their code when requirements change quickly.
Anti-patterns
Anti-patterns are design patterns you should avoid as they can introduce unnecessary complexity or create code that is hard to maintain. For example, the "God Object" anti-pattern occurs when developers try to cram too much functionality into a single object, creating an unmaintainable codebase.
The "Golden Hammer" anti-pattern can also occur when developers try to use the same solution for every problem without considering other options. That can lead to code that is difficult to understand and inefficient in its implementation.
Finally, the "Shotgun Surgery" anti-pattern occurs when developers change an existing application without adequately understanding its structure or considering how the changes may affect other components. This pattern can lead to unintended consequences and create a complicated codebase to maintain or debug.
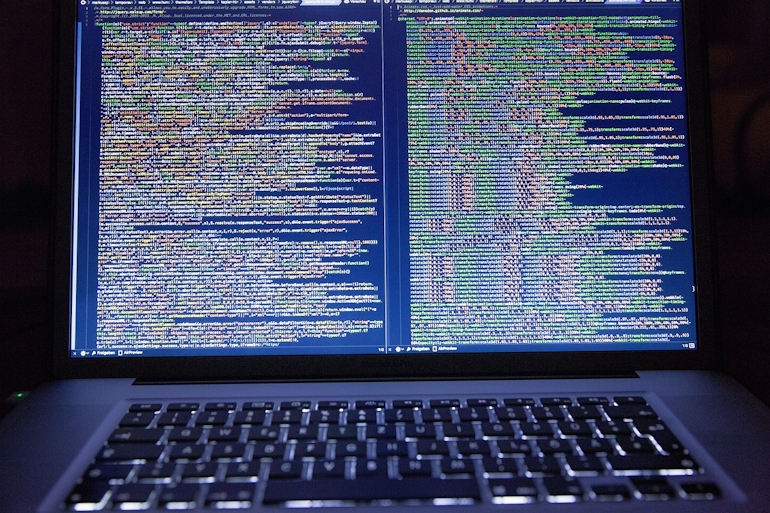
Best Practices for Using Software Design Patterns
Knowledge may not be enough to have a successful software development project. Combining that knowledge with some best practices is best to ensure that programmers can deliver reliable and efficient software.
Here are some of the best practices you can use with any of the software design patterns mentioned above:
Keep the code clean and maintainable
Maintaining clean and maintainable code is essential to any successful software development project. To keep the codebase organized and readable, developers should use descriptive variable names, follow consistent coding conventions, and refactor regularly.
Additionally, teams should strive to create modular code that can easily interact with other components, allowing for better scalability and reusability as the chain of responsibility gets passed on to new sets of developers.
Learn from Experience
Learning from real-world experience is an essential part of software development. By understanding the successes and failures of past projects, developers can identify potential pitfalls and uncover new ways to improve their designs.
Additionally, teams should strive to continually review and refine their codebase as they become more educated on best practices, such as adhering to established coding conventions and refraining from using obsolete ones.
Pro Tip
When using software design patterns, it's crucial to remember that they are not a silver bullet for every software development problem. Instead, they are tools that you should use with careful consideration for the problem at hand. Choose the correct pattern for the problem to ensure you are efficient with your software design.
Design Your Software With Brain Box Labs
Understanding each pattern's best practices and anti-patterns is critical to successful software design. With this knowledge, developers can create better code that is easier to maintain and debug.
Suppose you need help designing your software or want a partner to leverage these patterns in their development process. In that case, Brain Box Labs has experienced professionals ready to assist you every step of the way. Let us help you build a practical application by leveraging our expertise in software development.
Are you looking to take your software development to a whole new level? Brain Box Labs has a team of experts specializing in software development and computer science. Contact us today and learn how Brain Box Labs can help you.